前回の第26回
パーティクルの動き始めもランダムにする
前回できあがりの第26回コード4
var total = 200; // 100;
function resetParticle(particle) {
var lifetime = Math.random()* total | 0;
particle.reset(center.x, center.y, radius, angle, lifetime);
}
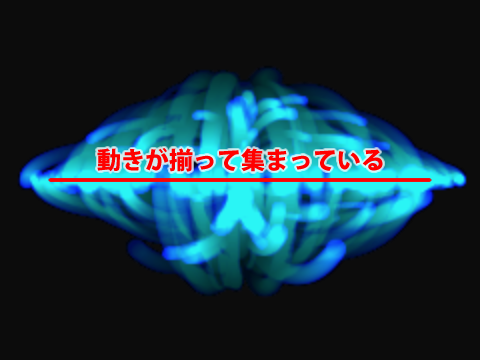
ここはやはり、
// Particle.prototype.reset = function(x, y, radius, angle, lifetime) {
Particle.prototype.reset = function(x, y, radius, angle, lifetime, delay) {
this.delay = delay;
this.index = 0;
};
Particle.prototype.isMovable = function() {
var movable = (++this.index > this.delay);
return movable;
};
アニメーションの関数
タメの値
function initialize() {
for(var i = 0; i < total; i++) {
// resetParticle(particle);
resetParticle(particle, i);
}
}
// function resetParticle(particle) {
function resetParticle(particle, delay) {
delay *= Math.random();
// particle.reset(center.x, center.y, radius, angle, lifetime);
particle.reset(center.x, center.y, radius, angle, lifetime, delay);
}
function tick() {
for(var i = 0; i < count; i++) {
var particle = particles[i];
// moveParticle(particle);
if (particle.isMovable()) {
moveParticle(particle, i);
}
}
}
// function moveParticle(particle) {
function moveParticle(particle, index) {
if (particle.lifetime > 0) {
} else {
// resetParticle(particle);
resetParticle(particle, index);
}
}
これらの書替えをおこなったパーティクルのクラス
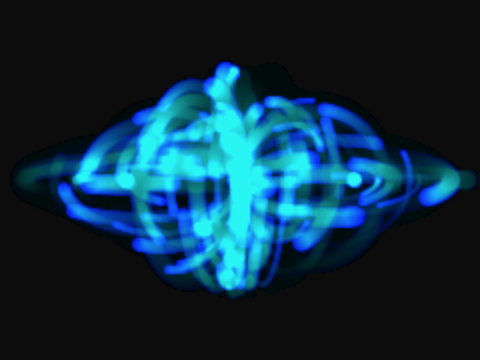
function Particle(radius, color) {
this.initialize();
this.graphics.beginFill(color)
.drawCircle(0, 0, radius)
.endFill();
this.compositeOperation = "lighter";
}
Particle.prototype = new createjs.Shape();
Particle.prototype.reset = function(x, y, radius, angle, lifetime, delay) {
this.x = x;
this.y = y;
this.speedX = radius * Math.cos(angle);
this.speedY = radius * Math.sin(angle);
this.lifetime = lifetime;
this.angle = 0;
this.delay = delay;
this.index = 0;
};
Particle.prototype.move = function(advance) {
var angle = this.angle + advance;
var velocityX = this.speedX * Math.sin(angle);
var velocityY = this.speedY * Math.cos(angle);
this.x += velocityX;
this.y += velocityY;
this.angle = angle;
this.lifetime--;
};
Particle.prototype.isMovable = function() {
var movable = (++this.index > this.delay);
return movable;
};
var stage;
var total = 200;
var center = new createjs.Point();
var particles = [];
var fading = 0.04;
function initialize() {
var canvasElement = document.getElementById("myCanvas");
var stageWidth = canvasElement.width;
var stageHeight = canvasElement.height;
stage = new createjs.Stage(canvasElement);
stage.autoClear = false;
center.x = stageWidth / 2;
center.y = stageHeight / 2;
for(var i = 0; i < total; i++) {
var radius = 1 + Math.random() * 4;
var particle = new Particle(radius, "#0016E9");
resetParticle(particle, i);
particles.push(particle);
stage.addChild(particle);
}
addBackground(stageWidth, stageHeight, fading);
createjs.Ticker.timingMode = createjs.Ticker.RAF;
createjs.Ticker.addEventListener("tick", tick);
}
function resetParticle(particle, delay) {
var radius = 1 + Math.random();
var angle = Math.random() * Math.PI * 2;
var lifetime = Math.random()* total | 0;
delay *= Math.random();
particle.reset(center.x, center.y, radius, angle, lifetime, delay);
}
function tick() {
var count = particles.length;
for(var i = 0; i < count; i++) {
var particle = particles[i];
if (particle.isMovable()) {
moveParticle(particle, i);
}
}
stage.update();
}
function moveParticle(particle, index) {
if (particle.lifetime > 0) {
particle.move(Math.PI / 90);
} else {
resetParticle(particle, index);
}
}
function addBackground(width, height, alpha) {
var background = new createjs.Shape();
background.graphics.beginFill("black")
.drawRect(0, 0, width, height)
.endFill();
stage.addChild(background);
background.alpha = alpha;
}
メソッドを動的に切換える
パーティクルのアニメーション表現そのものは、
Particle.prototype.isMovable = function() {
var movable = (++this.index > this.delay);
return movable;
};
function tick() {
if (particle.isMovable()) {
moveParticle(particle, i);
}
}
しかし、
function tick() {
// if (particle.isMovable()) {
moveParticle(particle, i);
// }
}
function moveParticle(particle, index) {
particle.move(Math.PI / 90);
}
さて、
そして、
Particle.prototype.reset = function(x, y, radius, angle, lifetime, delay) {
this.move = this._movable;
};
// Particle.prototype.move = function(advance) {
Particle.prototype._move = function(advance) {
};
// Particle.prototype.isMovable = function() {
Particle.prototype._movable = function(advance) {
// var movable = (++this.index > this.delay);
if (++this.index > this.delay) {
this.move = this._move;
}
// return movable;
};
これらの手直しをしたパーティクルのクラスの定めとそれを用いたJavaScriptコードは、
function Particle(radius, color) {
this.initialize();
this.graphics.beginFill(color)
.drawCircle(0, 0, radius)
.endFill();
this.compositeOperation = "lighter";
}
Particle.prototype = new createjs.Shape();
Particle.prototype.reset = function(x, y, radius, angle, lifetime, delay) {
this.x = x;
this.y = y;
this.speedX = radius * Math.cos(angle);
this.speedY = radius * Math.sin(angle);
this.lifetime = lifetime;
this.angle = 0;
this.delay = delay;
this.index = 0;
this.move = this._movable;
};
Particle.prototype._move = function(advance) {
var angle = this.angle + advance;
var velocityX = this.speedX * Math.sin(angle);
var velocityY = this.speedY * Math.cos(angle);
this.x += velocityX;
this.y += velocityY;
this.angle = angle;
this.lifetime--;
};
Particle.prototype._movable = function(advance) {
if (++this.index > this.delay) {
this.move = this._move;
}
};
var stage;
var total = 200;
var center = new createjs.Point();
var particles = [];
var fading = 0.04;
function initialize() {
var canvasElement = document.getElementById("myCanvas");
var stageWidth = canvasElement.width;
var stageHeight = canvasElement.height;
stage = new createjs.Stage(canvasElement);
stage.autoClear = false;
center.x = stageWidth / 2;
center.y = stageHeight / 2;
for(var i = 0; i < total; i++) {
var radius = 1 + Math.random() * 4;
var particle = new Particle(radius, "#0016E9");
resetParticle(particle, i);
particles.push(particle);
stage.addChild(particle);
}
addBackground(stageWidth, stageHeight, fading);
createjs.Ticker.timingMode = createjs.Ticker.RAF;
createjs.Ticker.addEventListener("tick", tick);
}
function resetParticle(particle, delay) {
var radius = 1 + Math.random();
var angle = Math.random() * Math.PI * 2;
var lifetime = Math.random()* total | 0;
delay *= Math.random();
particle.reset(center.x, center.y, radius, angle, lifetime, delay);
}
function tick() {
var count = particles.length;
for(var i = 0; i < count; i++) {
var particle = particles[i];
moveParticle(particle, i);
}
stage.update();
}
function moveParticle(particle, index) {
if (particle.lifetime > 0) {
particle.move(Math.PI / 90);
} else {
resetParticle(particle, index);
}
}
function addBackground(width, height, alpha) {
var background = new createjs.Shape();
background.graphics.beginFill("black")
.drawRect(0, 0, width, height)
.endFill();
stage.addChild(background);
background.alpha = alpha;
}
等速円運動と速度
結びとして、
現行角度 += 角速度
水平速度 = -半径×角速度×sin(現行角度)
垂直速度 = 半径×角速度×cos(現行角度)
水平座標(x) += 水平速度
垂直座標(y) += 垂直速度
速度とは、
速度 = 定数
位置 += 速度
等速直線運動でない場合、
速度 = 重力加速度×時間
位置 += 速度
数学では、
x = at2
v = x' = 2at
第25回
x = r cos(ωt)
y = r sin(ωt)
これらの方程式を微分すれば速度
x' = -rω sin(ωt)
y' = rω cos(ωt)
時間の単位をフレームとし
速度 += フレームあたりの加速度
位置 += 速度