前回は、
矩形のビットマップを任意の四角形に変形する
まずは、
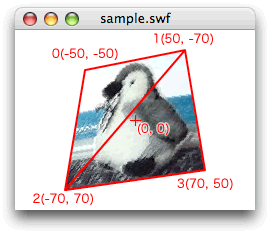
Graphics.
Graphicsオブジェクト.drawTriangles(頂点座標, null, uv座標)
// フレームアクション
var nCenterX:Number = stage.stageWidth / 2;
var nCenterY:Number = stage.stageHeight / 2;
var myShape:Shape = new Shape();
var myGraphics:Graphics = myShape.graphics;
var myTexture:BitmapData = new Image();
var vertices:Vector.<Number> = new Vector.<Number>();
var uvData:Vector.<Number> = new Vector.<Number>();
myShape.x = nCenterX;
myShape.y = nCenterY;
vertices.push(-50, -50); // 頂点0
vertices.push(50, -70); // 頂点1
vertices.push(-70, 70); // 頂点2
vertices.push(50, -70); // 頂点1 (重複)
vertices.push(70, 50); // 頂点3
vertices.push(-70, 70); // 頂点2 (重複)
uvData.push(0, 0);
uvData.push(1, 0);
uvData.push(0, 1);
uvData.push(1, 0); // 重複
uvData.push(1, 1);
uvData.push(0, 1); // 重複
myGraphics.beginBitmapFill(myTexture);
myGraphics.drawTriangles(vertices, null, uvData);
myGraphics.endFill();
addChild(myShape);
[ムービープレビュー]を確かめると、
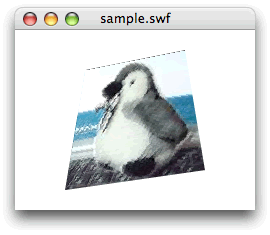
テクスチャマッピングは、
三角形の頂点の組を定める - Graphics.drawTriangles()メソッドの第2引数
前掲スクリプト1のGraphics.
Graphics.
// フレームアクション
var nCenterX:Number = stage.stageWidth / 2;
var nCenterY:Number = stage.stageHeight / 2;
var myShape:Shape = new Shape();
var myGraphics:Graphics = myShape.graphics;
var myTexture:BitmapData = new Image();
var vertices:Vector.<Number> = new Vector.<Number>();
var indices:Vector.<int> = new Vector.<int>();
var uvData:Vector.<Number> = new Vector.<Number>();
myShape.x = nCenterX;
myShape.y = nCenterY;
// 頂点座標
vertices.push(-50, -50); // 頂点0
vertices.push(50, -70); // 頂点1
vertices.push(-70, 70); // 頂点2
vertices.push(70, 50); // 頂点3
// 頂点番号
indices.push(0, 1, 2); // 左上三角形
indices.push(1, 3, 2); // 右下三角形
// uv座標
uvData.push(0, 0);
uvData.push(1, 0);
uvData.push(0, 1);
uvData.push(1, 1);
myGraphics.beginBitmapFill(myTexture);
myGraphics.drawTriangles(vertices, indices, uvData);
myGraphics.endFill();
addChild(myShape);
Graphics.
そして、
[ムービープレビュー]を確かめると、
描画する三角形の座標をマウスクリックで動かす
今回の最後は、
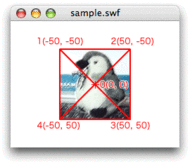
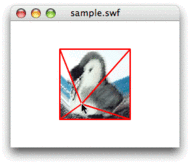
Shapeインスタンスはマウスクリックを受け取れない。そこで、
// フレームアクション
var nCenterX:Number = stage.stageWidth / 2;
var nCenterY:Number = stage.stageHeight / 2;
var mySprite:Sprite = new Sprite();
var myGraphics:Graphics = mySprite.graphics;
var myTexture:BitmapData = new Image();
var nHalfWidth:Number = myTexture.width / 2;
var nHalfHeight:Number = myTexture.height / 2;
var vertices:Vector.<Number> = new Vector.<Number>();
var indices:Vector.<int> = new Vector.<int>();
var uvData:Vector.<Number> = new Vector.<Number>();
mySprite.x = nCenterX;
mySprite.y = nCenterY;
// 頂点座標
vertices.push(0, 0); // 頂点0: マウスクリックで動かす座標
vertices.push(-nHalfWidth, -nHalfHeight);
vertices.push(nHalfWidth, -nHalfHeight);
vertices.push(nHalfWidth, nHalfHeight);
vertices.push(-nHalfWidth, nHalfHeight);
// 頂点番号
indices.push(0, 1, 2);
indices.push(0, 2, 3);
indices.push(0, 3, 4);
indices.push(0, 4, 1);
// uv座標
uvData.push(0.5, 0.5);
uvData.push(0, 0);
uvData.push(1, 0);
uvData.push(1, 1);
uvData.push(0, 1);
myGraphics.beginBitmapFill(myTexture);
myGraphics.drawTriangles(vertices, indices, uvData);
myGraphics.endFill();
addChild(mySprite);
mySprite.addEventListener(MouseEvent.MOUSE_DOWN, xDraw);
function xDraw(eventObject:MouseEvent):void {
// 頂点0の数値エレメント書替え
vertices[0] = eventObject.localX;
vertices[1] = eventObject.localY;
myGraphics.clear();
myGraphics.beginBitmapFill(myTexture);
myGraphics.drawTriangles(vertices, indices, uvData);
myGraphics.endFill();
}
Spriteインスタンスをマウスクリック
[ムービープレビュー]でSpriteインスタンスをクリックすると、
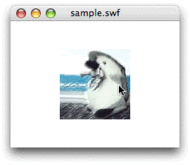
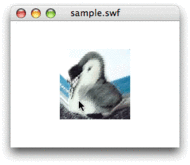
ようやく次回から、
今回解説した次のサンプルファイルがダウンロードできます。
スクリプト1~3のサンプルファイル(CS5形式/約106KB)