はじめに
Windows Phoneは、
Windows Phone OS 7.
今回はSNSの代表格でもあるFacebookの機能を利用するためのライブラリ
- FacebookTest.
zip (415KB)
Facebookのアプリケーション登録
FacebookのAPIを使用するには、
Facebook開発者サイトを開くと、
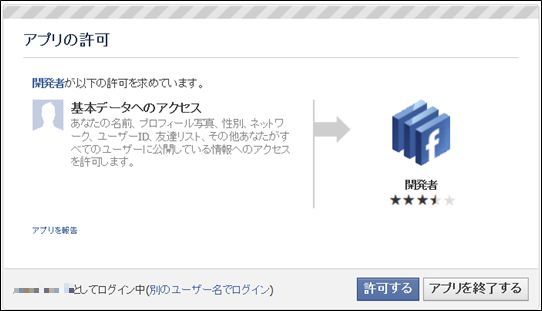
右上に表示されている
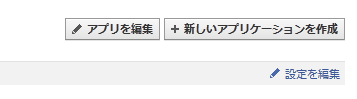
新しいアプリケーションの登録ダイアログが表示されるので、
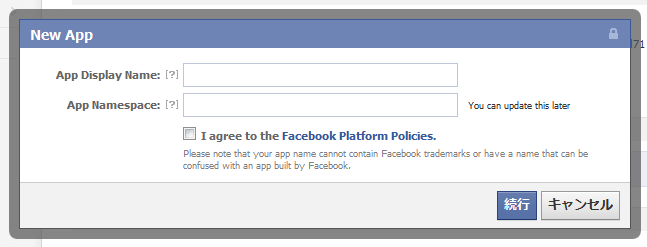
次に基本設定画面が表示されるので、
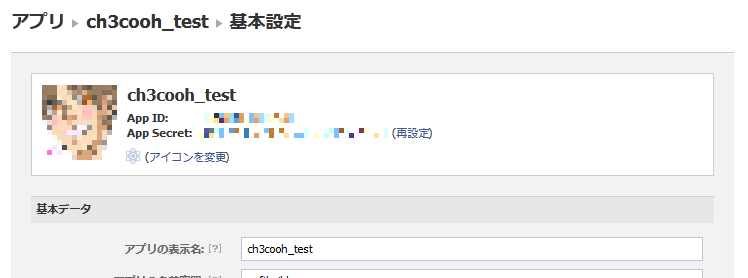
Windows PhoneアプリケーションとFacebookの連携を行うために、
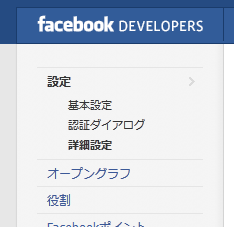
アプリケーションキーとシークレットキーを使ってユーザー認証を行うと、
認証自体はWindows Phoneアプリケーションで行うのですが、
その他項目に関しても下図を参照して頂いて、
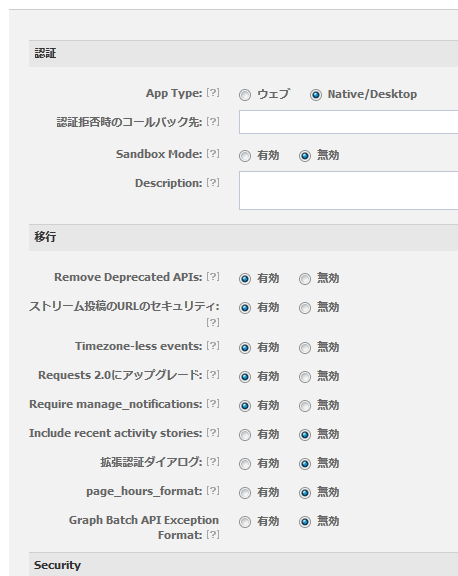
以上でFacebookへのアプリケーション登録準備は完了です。
Facebook C# SDKの導入
さて、
「Facebook C# SDK」
ここでは別段取り上げませんが、
右にダウンロードページへのリンクがありますので、
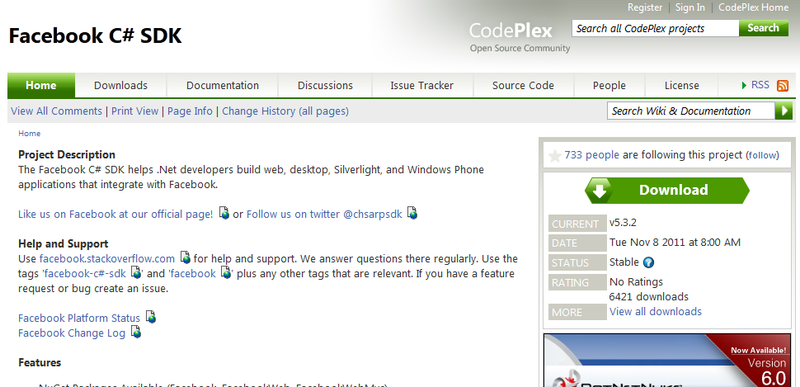
アセンブリファイルはzip形式で提供されていますので、
- Facebook.
dll - Facebook.
pdb - Facebook.
xml
後ほど、
ユーザー認証は、
この認証の一連のやりとりはWebBrowserコントロールで実施する必要があるので、
プロジェクトを新規に作成しましょう。プロジェクト名は
XAML
Facebookのログインに必要なのはウェブブラウザだけですので、
<phone:WebBrowser Name="webBrowser" Navigated="webBrowser_Navigated" Grid.ColumnSpan="2" IsScriptEnabled="True" />
取得したアクセストークンやログインしたユーザー名を表示させるTextBlockも準備しておきます。ユーザー名を表示するためのTextBlockやApplicationBarIconButtonに関しては次回使用します。
<phone:PhoneApplicationPage
x:Class="FacebookTest.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:phone="clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone"
xmlns:shell="clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d" d:DesignWidth="480" d:DesignHeight="696"
FontFamily="{StaticResource PhoneFontFamilyNormal}"
FontSize="{StaticResource PhoneFontSizeNormal}"
Foreground="{StaticResource PhoneForegroundBrush}"
SupportedOrientations="Portrait" Orientation="Portrait"
shell:SystemTray.IsVisible="True">
<Grid x:Name="LayoutRoot" Background="Transparent">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition/>
</Grid.RowDefinitions>
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock x:Name="ApplicationTitle" Text="SOFTBUILD" Style="{StaticResource PhoneTextNormalStyle}"/>
<TextBlock x:Name="PageTitle" Text="FacebookTest" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}"/>
</StackPanel>
<Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="0.329*"/>
<ColumnDefinition Width="0.671*"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="0.8*"/>
<RowDefinition Height="0.1*"/>
<RowDefinition Height="0.1*"/>
</Grid.RowDefinitions>
<phone:WebBrowser Name="webBrowser" Navigated="webBrowser_Navigated" Grid.ColumnSpan="2" IsScriptEnabled="True" />
<TextBlock Grid.Row="1" TextWrapping="Wrap" Text="AccessToken" d:LayoutOverrides="Width" VerticalAlignment="Center" Margin="12,0,0,0"/>
<TextBlock Grid.Row="2" TextWrapping="Wrap" Text="Name" VerticalAlignment="Center" Margin="12,0,0,0"/>
<TextBlock x:Name="textAccessToken" HorizontalAlignment="Left" Grid.Row="1" TextWrapping="Wrap" VerticalAlignment="Center" Margin="8,0,0,0" Grid.Column="1"/>
<TextBlock x:Name="textName" HorizontalAlignment="Left" Grid.Row="2" TextWrapping="Wrap" VerticalAlignment="Center" Margin="8,0,0,0" Grid.Column="1"/>
</Grid>
</Grid>
<phone:PhoneApplicationPage.ApplicationBar>
<shell:ApplicationBar IsVisible="True" IsMenuEnabled="True">
<shell:ApplicationBarIconButton IconUri="/icons/appbar.share.rest.png" Text="Update Statues"/>
<shell:ApplicationBarIconButton IconUri="/icons/appbar.upload.rest.png" Text="Upload"/>
</shell:ApplicationBar>
</phone:PhoneApplicationPage.ApplicationBar>
</phone:PhoneApplicationPage>
以上で、
C#
引き続き、
画面への突入時に呼ばれるOnNavigatedToメソッドにて、
下記のコード内にstring型の変数ApplicationIDとApplicationSecretを用意していますので、
using System.Collections.Generic;
using System.Diagnostics;
using System.Windows.Navigation;
using Facebook;
using Microsoft.Phone.Controls;
namespace FacebookTest {
public partial class MainPage : PhoneApplicationPage {
public MainPage() {
InitializeComponent();
}
// アプリケーション キー
private readonly string ApplicationID = "取得したアプリキー";
private readonly string ApplicationSecret = "取得したシークレットキー";
// アクセストークンを保持する
string accessToken = "";
protected override void OnNavigatedTo(System.Windows.Navigation.NavigationEventArgs e) {
var oauthClient = new FacebookOAuthClient { AppId = ApplicationID };
var prams = new Dictionary<string, object>();
prams["response_type"] = "code";
prams["scope"] = "user_about_me,user_photos,offline_access";
// 認証用のページURLを取得
var url = oauthClient.GetLoginUrl(prams);
// 認証ページへ遷移
webBrowser.Navigate(url);
}
先ほどの2つの正しいキーを設定し、
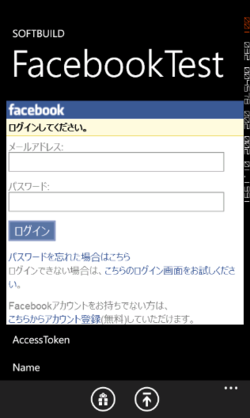
通常時にFacebookにアクセスする時のメールアドレスとパスワードを入力して、
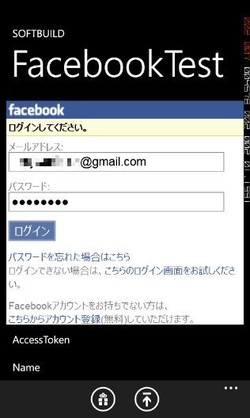
ユーザーのログインが完了後、
FacebookOAuthResult.
private void webBrowser_Navigated(object sender, NavigationEventArgs e) {
Debug.WriteLine("webBrowser_Navigated: {0}", e.Uri);
FacebookOAuthResult oauthResult;
if (!FacebookOAuthResult.TryParse(e.Uri, out oauthResult) || !oauthResult.IsSuccess) {
return;
}
var oauthClient = new FacebookOAuthClient { AppId = ApplicationID };
oauthClient.AppSecret = ApplicationSecret;
var code = oauthResult.Code;
// アクセストークンを要求(非同期実行)
oauthClient.ExchangeCodeForAccessTokenCompleted += oauthClient_ExchangeCodeForAccessTokenCompleted;
oauthClient.ExchangeCodeForAccessTokenAsync(code);
}
アクセストークンの取得処理が、
"access_
// アクセストークンを取得
void oauthClient_ExchangeCodeForAccessTokenCompleted(object sender, FacebookApiEventArgs e) {
var result = e.GetResultData() as IDictionary<string, object>;
if (result == null) return;
accessToken = (string)result["access_token"];
Dispatcher.BeginInvoke(() => {
textAccessToken.Text = accessToken;
});
}
}
}
おわりに
今回はFacebookでのアプリケーション登録から、
次回はアクセストークンを使ってFacebookのAPIを利用したいと思います。
今回は以上で終わりです。ありがとうございました。