前回の第46回は、
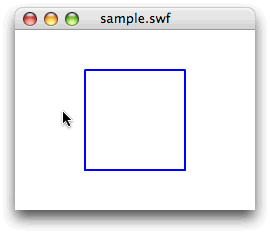
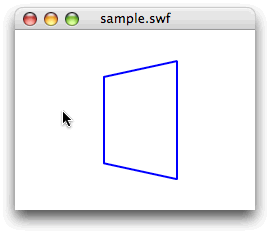
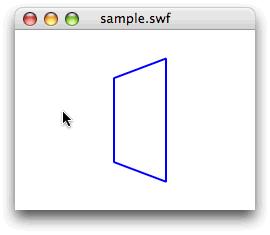
3次元空間で水平回転する正方形の頂点座標にテクスチャマッピングする
第43回スクリプト1が
[ライブラリ]
![第45回図1 塗りで使う[ライブラリ]のビットマップにクラス名「Image」を設定(再掲) 第45回図1 塗りで使う[ライブラリ]のビットマップにクラス名「Image」を設定(再掲)](/assets/images/dev/serial/01/as3/0047/004.gif)
それでは、
加えた内容は大きくふたつある
var nUnit:Number = 100 / 2;
var mySprite:Sprite = new Sprite();
var myTexture:BitmapData = new Image(); // 追加: ビットマップのインスタンス生成
var vertices:Vector.<Vector3D> = new Vector.<Vector3D>();
// 追加: Graphics.drawTriangles()メソッドに渡すVectorオブジェクト
var indices:Vector.<int> = new Vector.<int>(); // 頂点番号(第2引数)
var uvData:Vector.<Number> = new Vector.<Number>(); // uv座標(第3引数)
var nDeceleration:Number = 0.3;
var myGraphics:Graphics = mySprite.graphics;
var nFocalLength:Number = transform.perspectiveProjection.focalLength;
mySprite.x = stage.stageWidth / 2;
mySprite.y = stage.stageHeight / 2;
vertices.push(new Vector3D(-nUnit, -nUnit, 0));
vertices.push(new Vector3D(nUnit, -nUnit, 0));
vertices.push(new Vector3D(nUnit, nUnit, 0));
vertices.push(new Vector3D(-nUnit, nUnit, 0));
// 追加: 頂点番号の組
indices.push(0, 1, 3);
indices.push(1, 2, 3);
// 追加: uv座標値
uvData.push(0, 0);
uvData.push(1, 0);
uvData.push(1, 1);
uvData.push(0, 1);
addChild(mySprite);
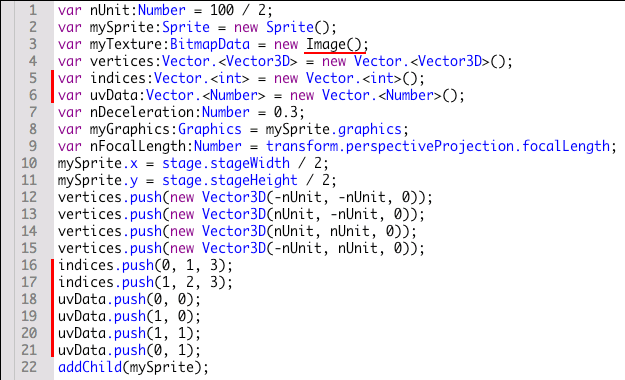
つぎは、
addEventListener(Event.ENTER_FRAME, xRotate);
function xRotate(eventObject:Event):void {
var nRotationY:Number = mySprite.mouseX * nDeceleration;
xTransform(vertices, nRotationY);
// var vertices2D:Vector.<Point> = xGetVertices2D(vertices);
var vertices2D:Vector.<Number> = xGetVertices2D(vertices); // 変更: ベース型をNumberに
// xDrawLines(vertices2D);
xDraw(vertices2D); // 変更: テクスチャマッピングの関数を呼出す
}
そして、
// function xGetVertices2D(myVertices:Vector.<Vector3D>):Vector.<Point> {
function xGetVertices2D(myVertices:Vector.<Vector3D>):Vector.<Number> {
// var vertices2D:Vector.<Point> = new Vector.<Point>();
var vertices2D:Vector.<Number> = new Vector.<Number>();
var nLength:uint = myVertices.length;
for (var i:uint = 0; i < nLength; i++) {
var myVector3D:Vector3D = myVertices[i].clone();
myVector3D.w = (nFocalLength + myVector3D.z) / nFocalLength;
myVector3D.project();
// vertices2D.push(new Point(myVector3D.x, myVector3D.y));
vertices2D.push(myVector3D.x, myVector3D.y);
}
return vertices2D;
}
最後に、
/* 削除
function xDrawLines(vertices2D:Vector.<Point>):void {
// ...[中略]...
}
*/
function xDraw(vertices2D:Vector.<Number>):void {
myGraphics.clear();
myGraphics.beginBitmapFill(myTexture);
myGraphics.drawTriangles(vertices2D, indices, uvData);
myGraphics.endFill();
}
でき上がったフレームアクションの全体は、
// フレームアクション
var nUnit:Number = 100 / 2;
var mySprite:Sprite = new Sprite();
var myTexture:BitmapData = new Image();
var vertices:Vector.<Vector3D> = new Vector.<Vector3D>();
var indices:Vector.<int> = new Vector.<int>();
var uvData:Vector.<Number> = new Vector.<Number>();
var nDeceleration:Number = 0.3;
var myGraphics:Graphics = mySprite.graphics;
var nFocalLength:Number = transform.perspectiveProjection.focalLength;
mySprite.x = stage.stageWidth / 2;
mySprite.y = stage.stageHeight / 2;
vertices.push(new Vector3D(-nUnit, -nUnit, 0));
vertices.push(new Vector3D(nUnit, -nUnit, 0));
vertices.push(new Vector3D(nUnit, nUnit, 0));
vertices.push(new Vector3D(-nUnit, nUnit, 0));
indices.push(0, 1, 3);
indices.push(1, 2, 3);
uvData.push(0, 0);
uvData.push(1, 0);
uvData.push(1, 1);
uvData.push(0, 1);
addChild(mySprite);
addEventListener(Event.ENTER_FRAME, xRotate);
function xRotate(eventObject:Event):void {
var nRotationY:Number = mySprite.mouseX * nDeceleration;
xTransform(vertices, nRotationY);
var vertices2D:Vector.<Number> = xGetVertices2D(vertices);
xDraw(vertices2D);
}
function xTransform(myVertices:Vector.<Vector3D>, myRotation:Number):void {
var nLength:uint = myVertices.length;
var myMatrix3D:Matrix3D = new Matrix3D();
myMatrix3D.prependRotation(myRotation, Vector3D.Y_AXIS);
for (var i:int = 0; i<nLength; i++) {
myVertices[i] = myMatrix3D.transformVector(myVertices[i]);
}
}
function xGetVertices2D(myVertices:Vector.<Vector3D>):Vector.<Number> {
var vertices2D:Vector.<Number> = new Vector.<Number>();
var nLength:uint = myVertices.length;
for (var i:uint = 0; i < nLength; i++) {
var myVector3D:Vector3D = myVertices[i].clone();
myVector3D.w = (nFocalLength + myVector3D.z) / nFocalLength;
myVector3D.project();
vertices2D.push(myVector3D.x, myVector3D.y);
}
return vertices2D;
}
function xDraw(vertices2D:Vector.<Number>):void {
myGraphics.clear();
// myGraphics.lineStyle(1, 0x0000FF); // 確認用
myGraphics.beginBitmapFill(myTexture);
myGraphics.drawTriangles(vertices2D, indices, uvData);
myGraphics.endFill();
}
テクスチャがゆがむ謎
前掲スクリプト1のキャプションに
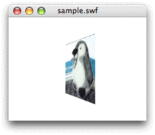
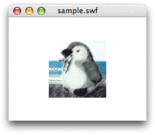
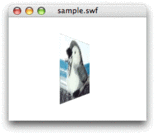
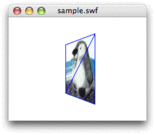
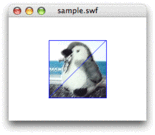
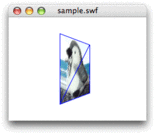
前掲スクリプト1の関数xDraw()内に、
myGraphics.lineStyle(1, 0x0000FF); // 確認用
とくに画像中程の水平線に注目すると、
正方形を回してゆがみを確かめると、
テクスチャに遠近法の適用なし
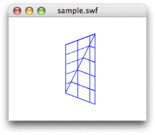
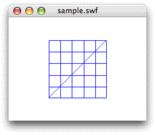
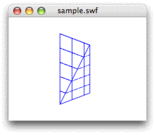
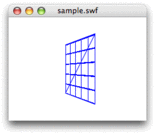
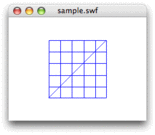
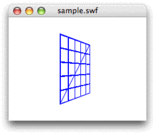
前掲スクリプト1は、
今回解説した次のサンプルファイルがダウンロードできます。
- スクリプト1のサンプルファイル
(CS5形式/約37KB)